[Flutter] 매우 쉽게 Flutter 푸시 메세지(FCM) 사용하기
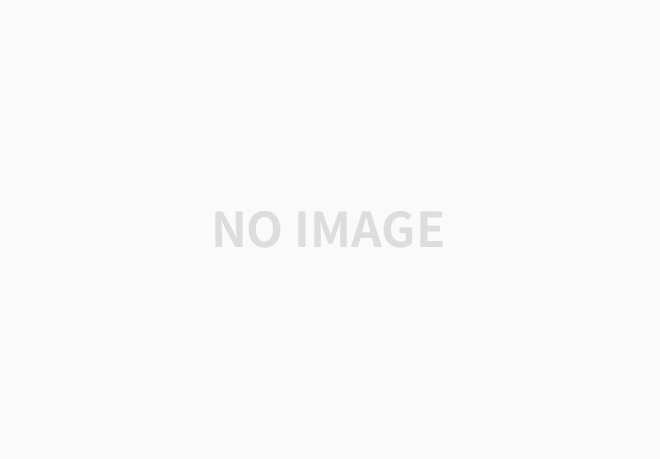
'AWS로 구현하는 MAS와 컨테이너 오캐스트레이션' 강의를
블로그를 통해 구매하시는 분들에게만 10%할인 중입니다.
이미지를 클릭하면 강의로 이동할 수 있습니다.
아래 쿠폰번호를 입력해 주세요!
16861-259843d6c2d7
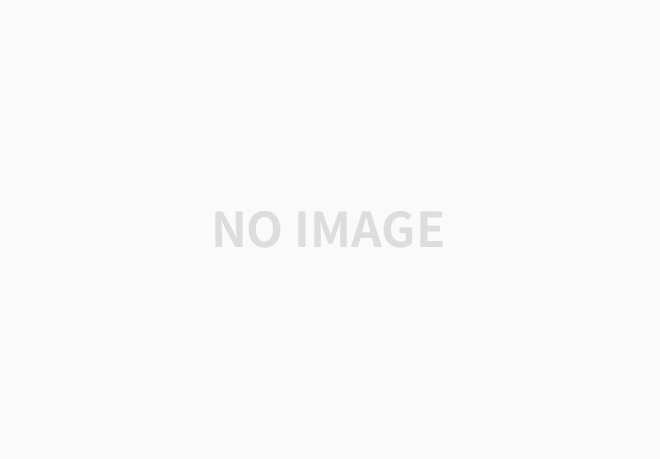
시작 전 프로젝트에 firebase 설정
[FlutterFire] 아주 쉽게 Flutter프로젝트 Firebase 설정 (with firebase CLI)
Firebase CLI 설치 및 로그인 curl -sL https://firebase.tools | bash 위 명령어를 입력하고 password를 입력하면 cli가 설치된다. version 명령어를 통해 설치가 되었는지 확인 firebase login firebase logi..
kanoos-stu.tistory.com
FCM 라이브러리 추가
flutter pub add firebase_messaging
위의 명령어를 통해 자신의 flutter 버전에 맞는 fcm 라이브러리를 추가한다.
firebase_messaging | Flutter Package
Flutter plugin for Firebase Cloud Messaging, a cross-platform messaging solution that lets you reliably deliver messages on Android and iOS.
pub.dev
flutter pub add flutter_local_notifications
포그라운드 수신 메시지의 푸시를 출력하는 라이브러리를 추가한다.
flutter_local_notifications | Flutter Package
A cross platform plugin for displaying and scheduling local notifications for Flutter applications with the ability to customise for each platform.
pub.dev
FCM 초기화 및 메세지 수신 설정
FCM 토큰 생성
기기의 FCM토큰을 생성하기 위해 firebase 프로젝트의 웹 푸시 인증키가 필요하다.
Firebase 콘솔 -> 해당 프로젝트 -> 프로젝트 설정 -> '클라우드 메시징' 탭 -> 하단의 웹 구성에서 웹 푸시 인증서 생성 -> 키 쌍 복사
var fcmToken = await FirebaseMessaging.instance.getToken(vapidKey: "firebase 웹 푸시 인증 키");
// save token to server
위의 코드를 통해 해당 기기의 FCM토큰을 발급받을 수 있다.
FCM 새로고침 수신
FirebaseMessaging.instance.onTokenRefresh.listen((newToken) async {
// save token to server
});
FCM 토큰은 사용자가 삭제, 재설치 및 앱 데이터를 지우면 새로고침이 되어 기존의 토큰은 효력이 없어지고 새로은 토큰이 발급된다.
위 코드를 통해 새로 발급된 토큰의 스트림을 받을 수 있다.
기존 FCM 토큰 삭제
FirebaseMessaging.instance.deleteToken();
기존에 발급받았던 토큰을 삭제
FCM 초기화 및 백그라운드 메세지 수신
// main.dart
Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async {
await Firebase.initializeApp();
print("Handling a background message: ${message.messageId}");
}
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(options: DefaultFirebaseOptions.currentPlatform);
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
runApp(const MyApp());
}
앱이 백그라운드 상태일때 수신되는 메세지를 처리하는 부분
iOS 권한 요청
Future requestPermission() async {
NotificationSettings settings = await _messaging.requestPermission(
alert: true,
announcement: false,
badge: true,
carPlay: false,
criticalAlert: false,
provisional: false,
sound: true,
);
}
- alert - 권한 요청 알림 화면을 표시 (default true)
- announcement - 시리를 통해 알림의 내용을 자동으로 읽을 수 있는 권한 요청 (default false)
- badge - 뱃지 업데이트 권한 요청 (default true)
- carPlay - carPlay 환경에서 알림 표시 권한 요청 ( default false)
- criticalAlert - 중요 알림에 대한 권한 요청, 해당 알림 권한을 요청하는 이유를 app store 등록시 명시해야함 (default true)
- provisional - 무중단 알림 권한 요청 (default false)
- sound - 알림 소리 권한 요청 (default true)
Android 알림 채널 설정
Android 버전 8(API 수준 26) 이상 부터는 채널설정이 필수이다.
var channel = const AndroidNotificationChannel(
'high_importance_channel', // id
'High Importance Notifications', // name
description: 'This channel is used for important notifications.', // description
importance: Importance.high,
);
- id(필수) - 채널 아이디 설정
- name(필수) - 채널 이름 설정
- description - 채널 설명
- groupId - 채널이 속한 그룹의 id
- importance - 알림의 중요도
- playSound - 알림 소리 여부 설정
- sound - 재생할 소리 지정, playSound가 true이어야함.
- enableVibration - 알림 진동 여부
- enableLights - 알림 조명 여부
- vibrationPattern - 알림 진동 패턴 설정
- ledColor - 알림 조명의 색상을 지정
- showBadge - 뱃지 표시 여부 지정
FCM 포그라운드 수신
var flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin();
await flutterLocalNotificationsPlugin
.resolvePlatformSpecificImplementation<AndroidFlutterLocalNotificationsPlugin>()
?.createNotificationChannel(channel);
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Got a message whilst in the foreground!');
print('Message data: ${message.data}');
if (message.notification != null) {
print('Message also contained a notification: ${message.notification}');
flutterLocalNotificationsPlugin.show(
message.hashCode,
message.notification?.title,
message.notification?.body,
NotificationDetails(
android: AndroidNotificationDetails(
channel.id,
channel.name,
channelDescription: channel.description,
icon: '@mipmap/ic_launcher',
),
iOS: const IOSNotificationDetails(
badgeNumber: 1,
subtitle: 'the subtitle',
sound: 'slow_spring_board.aiff',
)));
}
});
위의 코드를 통해 포그라운드에서의 푸시알림을 수신할 수 있으며 각각의 플랫폼별 설정은 AndroidNotificationDetails, IOSNotificationDetails 클래스를 통해 자세하게 설정할 수 있다.
FCM 백그라운드 메시지 클릭 액션
Future<void> setupInteractedMessage() async {
RemoteMessage? initialMessage = await FirebaseMessaging.instance.getInitialMessage();
// 종료상태에서 클릭한 푸시 알림 메세지 핸들링
if (initialMessage != null) _handleMessage(initialMessage);
// 앱이 백그라운드 상태에서 푸시 알림 클릭 하여 열릴 경우 메세지 스트림을 통해 처리
FirebaseMessaging.onMessageOpenedApp.listen(_handleMessage);
}
void _handleMessage(RemoteMessage message) {
print('message = ${message.notification!.title}');
if (message.data['type'] == 'chat') {
Get.toNamed('/chat', arguments: message.data);
}
}
- FirebaseMessaging.instance.getInitialMessage() - 앱이 종료된 상태에서 푸시 알림 클릭하여 열릴 경우 메세지 가져옴
- FirebaseMessaging.onMessageOpenApp.listen - 앱이 백그라운드 상태에서 푸시 알림 클릭하여 열릴 경우 메세지 스트림
FCM 포그라운드 메시지 클릭 액션
const AndroidInitializationSettings initializationSettingsAndroid = AndroidInitializationSettings('app_icon');
const IOSInitializationSettings initializationSettingsIOS = IOSInitializationSettings();
const InitializationSettings initializationSettings =
InitializationSettings(android: initializationSettingsAndroid, iOS: initializationSettingsIOS);
flutterLocalNotificationsPlugin.initialize(initializationSettings, onSelectNotification: (payload) {
if (payload != null) {
Get.to(const NextPage(), arguments: payload);
}
});
위 코드를 통해 수신된 메시지의 클릭 액션을 구현할 수 있다.
*참고
Firebase Cloud Messaging | FlutterFire
What does it do?
firebase.flutter.dev